Boost is a set of open source C++ libraries that build on the ISO C++ programming language. In some cases, the Boost library functionality has become part of recent ISO C++ standards. RAD Studio allows you to install a subset of Boost that has been fully tested and preconfigured specifically for C++Builder. Use the GetIt Package Manager to install the Boost libraries for the Win32 classic C++ compiler, Win32 Clang-enhanced C++ compiler and Win64 Clang-enhanced compiler.
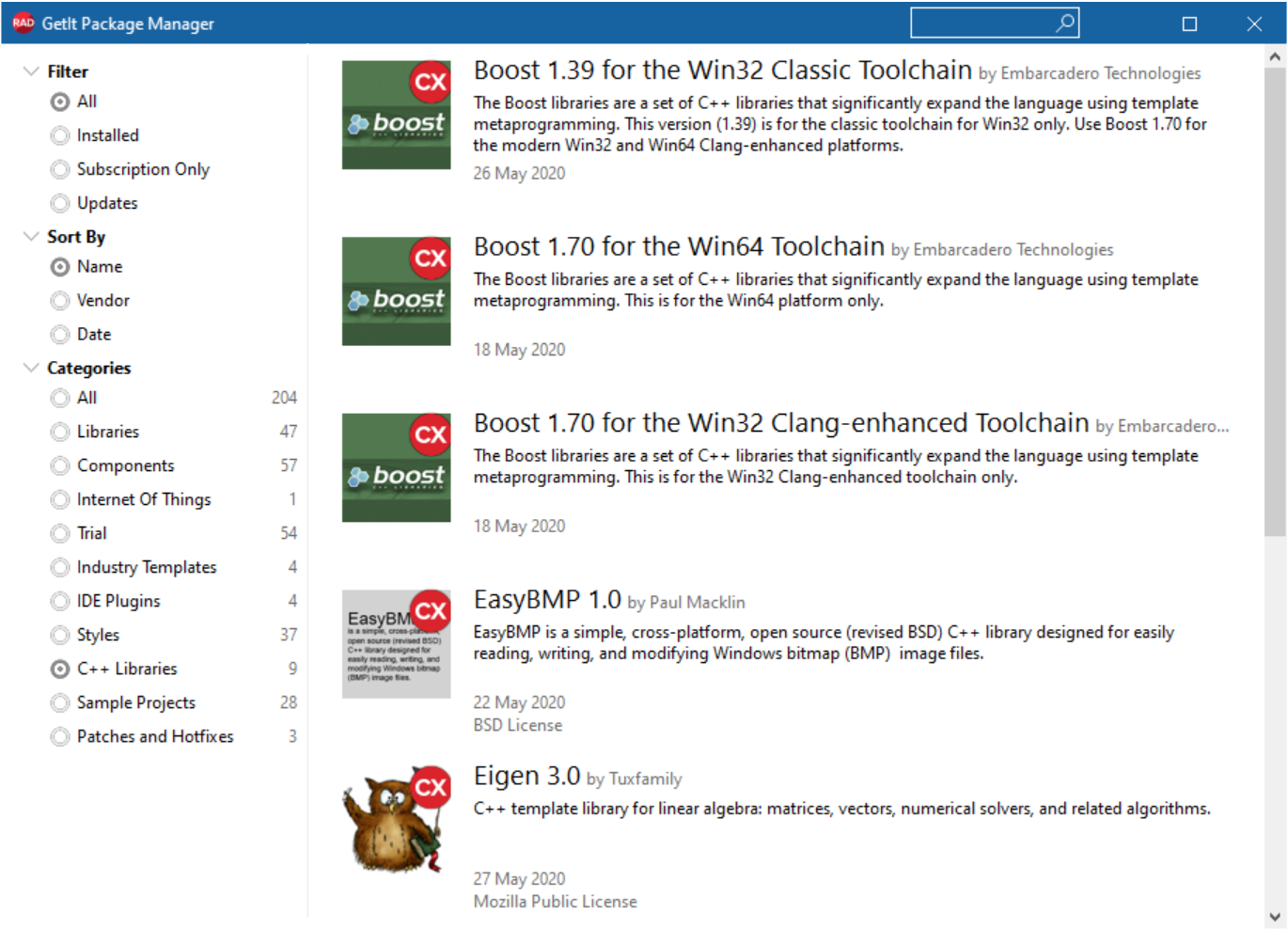
boost::filesystem and std:filesystem VCL example
The ISO C++ std::filesystem evolved from the Boost filesystem library. The Filesystem library started in Boost, then became an ISO C++ Technical Specification and was finally merged into the ISO C++17 standard. The first example shows how to create a C++Builder VCL application using the Boost filesystem and the ISO C++ filesystem.
The VCL form contains two TButton, one TEdit and two TMemo components. The TEdit is used to set a path to files and directories on your hard drive. One button OnClick event handler will use the boost::filesystem functions to display the contents of the path. The other button OnCLick event handler will use the std::filesystem functions to display the contents of the same path. Why use both libraries? You may have an application and compiler than does not support the latest C++17 filesystem library standard. There are additional boost library versions available to support a wider range of platform filesystem operations.
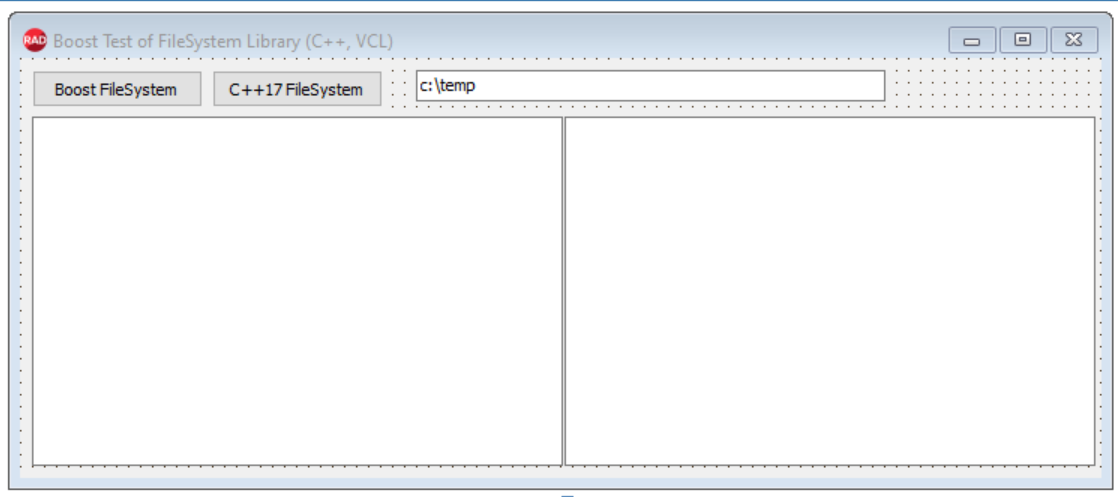
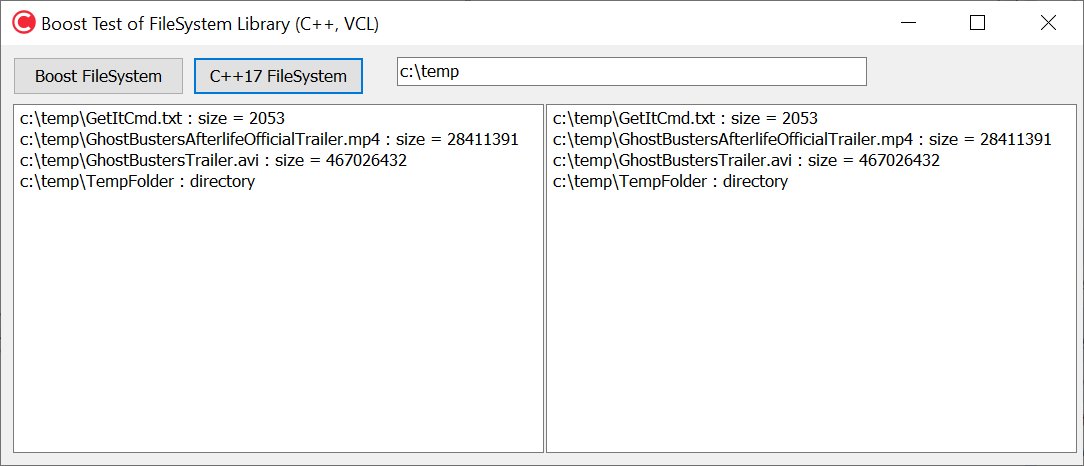
Filesystem VCL app mainunit.h: //--------------------------------------------------------------------------- #ifndef MainUnitH #define MainUnitH //--------------------------------------------------------------------------- #include <System.Classes.hpp> #include <Vcl.Controls.hpp> #include <Vcl.StdCtrls.hpp> #include <Vcl.Forms.hpp> //--------------------------------------------------------------------------- class TForm1 : public TForm { __published: // IDE-managed Components TButton *BoostButton; TEdit *Edit1; TMemo *Memo1; TButton *Cpp17Button; TMemo *Memo2; void __fastcall BoostButtonClick(TObject *Sender); void __fastcall Cpp17ButtonClick(TObject *Sender); private: // User declarations public: // User declarations __fastcall TForm1(TComponent* Owner); }; //--------------------------------------------------------------------------- extern PACKAGE TForm1 *Form1; //--------------------------------------------------------------------------- #endif Filesystem VCL App MainUnit.cpp: //--------------------------------------------------------------------------- #include <vcl.h> /* https://en.cppreference.com/w/cpp/filesystem The filesystem library was originally developed as boost.filesystem, was published as the technical specification ISO/IEC TS 18822:2015, and finally merged to ISO C++ as of C++17. https://www.boost.org/doc/libs/1_70_0/libs/filesystem/doc/index.htm */ #include <boost/filesystem.hpp> namespace Boostfs = boost::filesystem; #include <filesystem> namespace Cpp17fs = std::filesystem; #pragma hdrstop #include "MainUnit.h" //--------------------------------------------------------------------------- #pragma package(smart_init) #pragma resource "*.dfm" TForm1 *Form1; //--------------------------------------------------------------------------- __fastcall TForm1::TForm1(TComponent* Owner) : TForm(Owner) { } //--------------------------------------------------------------------------- void __fastcall TForm1::BoostButtonClick(TObject *Sender) { // Boost Filesystem verion Memo1->Lines->Clear(); // use the Boost FileSystem to get directories and files using path in editbox Boostfs::path directoryPath = Edit1->Text.c_str(); for (const auto& entry : Boostfs::directory_iterator(directoryPath)) { Boostfs::path p = entry.path(); // test if the path is a file if (is_regular_file(p)) { int fsize = file_size(p); std::string s = p.string() + " : size = "; Memo1->Lines->Add(s.c_str() + IntToStr(fsize)); } // test if the path is a directory else if (is_directory(p)) { // is p a directory? std::string s = p.string() + " : directory"; Memo1->Lines->Add(s.c_str()); } // otherwise it is something else :) else { std::string s = p.string() + " not a file or directory"; Memo1->Lines->Add(p.c_str()); } } } //--------------------------------------------------------------------------- void __fastcall TForm1::Cpp17ButtonClick(TObject *Sender) { // C++17 FileSystem version Memo2->Lines->Clear(); // use C++17 std FileSystem library to get directories given a path in the editbox Cpp17fs::path directoryPath = Edit1->Text.c_str(); for (const auto& entry : Cpp17fs::directory_iterator(directoryPath)) { Cpp17fs::path p = entry.path(); if (Cpp17fs::is_directory(p)) { std::string s = p.string() + " : directory"; Memo2->Lines->Add(s.c_str()); } else if (Cpp17fs::is_regular_file(p)) { int fsize = Cpp17fs::file_size(p); std::string s = p.string() + " : size = "; Memo2->Lines->Add(s.c_str() + IntToStr(fsize)); } else { std::string s = p.string() + " not a file or directory"; Memo2->Lines->Add(p.c_str()); } } } //---------------------------------------------------------------------------
boost::circular_buffer VCL example
The boost circular buffer (also known as a ring or cyclic buffer) library allows for the storing of data. The boost is designed to support fixed capacity storage. When the buffer is full, additional elements will overwrite existing elements at the front and back of the buffer (depending on the operations used).
The VCL form contains three TButton, one TSpinEdit and one TMemo components. One TButton OnClick event handler shows the contents of the circular buffer (originally populated by the Form’s OnShow event handler. The other two TButton OnClick event handlers use the boost circular buffer push_front and push_back public member functions.
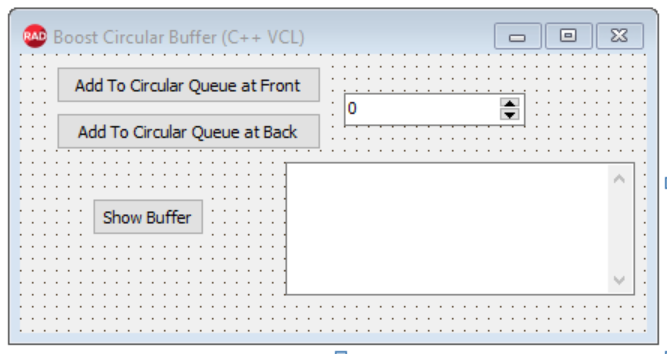
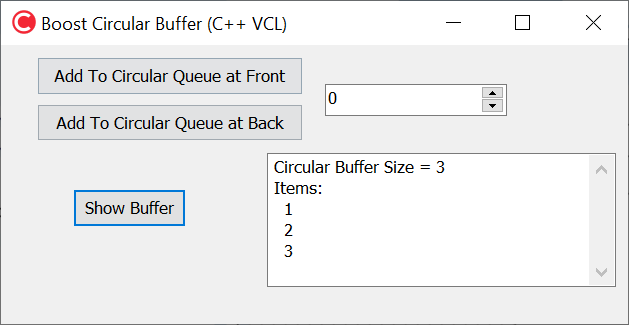
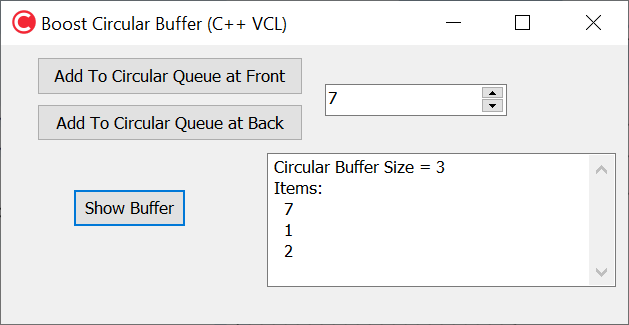
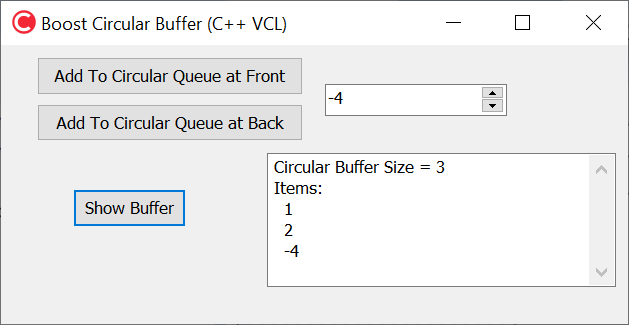
Circular Buffer VCL app mainunit.h: //--------------------------------------------------------------------------- #ifndef MainUnitH #define MainUnitH //--------------------------------------------------------------------------- #include <System.Classes.hpp> #include <Vcl.Controls.hpp> #include <Vcl.StdCtrls.hpp> #include <Vcl.Forms.hpp> #include <Vcl.Samples.Spin.hpp> //--------------------------------------------------------------------------- class TForm1 : public TForm { __published: // IDE-managed Components TButton *AddToCircularQueueFrontButton; TSpinEdit *SpinEdit1; TMemo *Memo1; TButton *ShowCircularBufferButton; TButton *AddToCircularQueueBackButton; void __fastcall FormShow(TObject *Sender); void __fastcall ShowCircularBufferButtonClick(TObject *Sender); void __fastcall AddToCircularQueueFrontButtonClick(TObject *Sender); void __fastcall AddToCircularQueueBackButtonClick(TObject *Sender); private: // User declarations public: // User declarations __fastcall TForm1(TComponent* Owner); }; //--------------------------------------------------------------------------- extern PACKAGE TForm1 *Form1; //--------------------------------------------------------------------------- #endif Circular Buffer VCL app mainunit.cpp: //--------------------------------------------------------------------------- #include <vcl.h> #include <boost/circular_buffer.hpp> #pragma hdrstop #include "MainUnit.h" //--------------------------------------------------------------------------- #pragma package(smart_init) #pragma resource "*.dfm" TForm1 *Form1; // Create a circular buffer with a capacity for 3 integers. boost::circular_buffer<int> cb(3); //--------------------------------------------------------------------------- __fastcall TForm1::TForm1(TComponent* Owner) : TForm(Owner) { } //--------------------------------------------------------------------------- void __fastcall TForm1::FormShow(TObject *Sender) { // initialize circular buffer with 3 integers cb.push_back(1); cb.push_back(2); cb.push_back(3); } //--------------------------------------------------------------------------- void __fastcall TForm1::ShowCircularBufferButtonClick(TObject *Sender) { int circularbuffersize = cb.size(); Memo1->Lines->Clear(); Memo1->Lines->Add("Circular Buffer Size = "+IntToStr(circularbuffersize)); Memo1->Lines->Add("Items:"); // display items in the circular buffer for (int i : cb) Memo1->Lines->Add(" "+IntToStr(i)); } //--------------------------------------------------------------------------- void __fastcall TForm1::AddToCircularQueueFrontButtonClick(TObject *Sender) { cb.push_front(SpinEdit1->Value); } //--------------------------------------------------------------------------- void __fastcall TForm1::AddToCircularQueueBackButtonClick(TObject *Sender) { cb.push_back(SpinEdit1->Value); } //---------------------------------------------------------------------------
References
Converting from Boost to std::filesystem by Scott Furry as a guest post on Bartlomiej Filipek (Bartek) blog.
boost::filesystem library documentation
C++ std::filesystem documentation
boost::circular_buffer documentation
boost and std filesystem VCL app source code project (zip file)
boost circular buffer VCL app source code project (zip file)
C++Builder Product Information
C++Builder Product Page – Native Apps that Perform. Build Windows C++ Apps 10x Faster with Less Code
C++Builder Product Editions – C++Builder is available in four editions – Professional, Enterprise, Architect and Community (free). C++Builder is also available as part of the RAD Studio development suite.